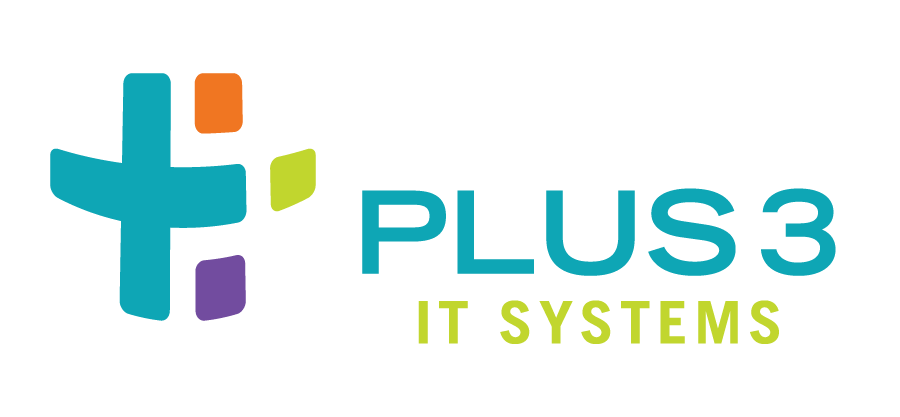
API Reference
watchmaker
Watchmaker module.
- class watchmaker.Arguments(config_path=None, log_dir=None, no_reboot=False, log_level=None, *args, **kwargs)[source]
Bases:
dict
Create an arguments object for the
watchmaker.Client
.- Parameters:
config_path – (
str
) Path or URL to the Watchmaker configuration file. IfNone
, the default config.yaml file is used. (Default:None
)log_dir – (
str
) Path to a directory. If set, Watchmaker logs to a file namedwatchmaker.log
in the specified directory. Both the directory and the file will be created if necessary. If the file already exists, Watchmaker appends to it rather than overwriting it. If this argument evaluates toFalse
, then logging to a file is disabled. Watchmaker will always output to stdout/stderr. Additionaly, Watchmaker workers may use this directory to keep other log files. (Default:None
)no_reboot – (
bool
) Switch to control whether to reboot the system upon a successful execution ofwatchmaker.Client.install()
. When this parameter is set, Watchmaker will suppress the reboot. Watchmaker automatically suppresses the reboot if it encounters an error. (Default:False
)log_level – (
str
) Level to log at. Case-insensitive. Valid options include, from least to most verbose:critical
error
warning
info
debug
Important
For all Keyword Arguments, below, the default value of
None
means Watchmaker will get the value from the configuration file. Be aware thatNone
and'None'
are two different values, with different meanings and effects.- Keyword Arguments:
admin_groups –
(
str
) Set a salt grain that specifies the domain _groups_ that should have root privileges on Linux or admin privileges on Windows. Value must be a colon-separated string. On Linux, use the^
to denote spaces in the group name. (Default:None
)admin_groups = "group1:group2" # (Linux only) The group names must be lowercased. Also, if # there are spaces in a group name, replace the spaces with a # '^'. admin_groups = "space^out" # (Windows only) No special capitalization nor syntax # requirements. admin_groups = "Space Out"
admin_users –
(
str
) Set a salt grain that specifies the domain _users_ that should have root privileges on Linux or admin privileges on Windows. Value must be a colon-separated string. (Default:None
)admin_users = "user1:user2"
computer_name – (
str
) Set a salt grain that specifies the computername to apply to the system. (Default:None
)environment – (
str
) Set a salt grain that specifies the environment in which the system is being built. For example:dev
,test
, orprod
. (Default:None
)salt_states – (
str
) Comma-separated string of salt states to apply. A value ofNone
will not apply any salt states. A value of'Highstate'
will apply the salt highstate. (Default:None
)ou_path –
(
str
) Set a salt grain that specifies the full DN of the OU where the computer account will be created when joining a domain. (Default:None
)ou_path="OU=Super Cool App,DC=example,DC=com"
extra_arguments –
(
list
) A list of extra arguments to be merged into the worker configurations. The list must be formed as pairs of named arguments and values. Any leading hypens in the argument name are stripped. (Default:[]
)extra_arguments=['--arg1', 'value1', '--arg2', 'value2'] # This list would be converted to the following dict and merged # into the parameters passed to the worker configurations: {'arg1': 'value1', 'arg2': 'value2'}
- class watchmaker.Client(arguments)[source]
Bases:
object
Prepare a system for setup and installation.
- Keyword Arguments:
arguments – (
Arguments
) A dictionary of arguments. Seewatchmaker.Arguments
.
watchmaker.managers
Watchmaker managers module.
watchmaker.managers.platform
Watchmaker base manager.
- class watchmaker.managers.platform.PlatformManagerBase(system_params, *args, **kwargs)[source]
Bases:
object
Base class for operating system managers.
All child classes will have access to methods unless overridden by an identically-named method in the child class.
- Parameters:
system_params – (
dict
) Attributes, mostly file-paths, specific to the system-type (Linux or Windows). The dict keys are as follows:- prepdir:
Directory where Watchmaker will keep files on the system.
- readyfile:
Path to a file that will be created upon successful completion.
- logdir:
Directory to store log files.
- workingdir:
Directory to store temporary files. Deleted upon successful completion.
- restart:
Command to use to restart the system upon successful completion.
- shutdown_path:
(Windows-only) Path to the Windows
shutdown.exe
command.
- retrieve_file(url, filename)[source]
Retrieve a file from a provided URL.
Supports all
urllib.request
handlers, as well as S3 buckets.
- call_process(cmd, log_pipe='all', raise_error=True)[source]
Execute a shell command.
- extract_contents(filepath, to_directory, create_dir=False)[source]
Extract a compressed archive to the specified directory.
- Parameters:
filepath – (
str
) Path to the compressed file. Supported file extensions:.zip
.tar.gz
.tgz
.tar.bz2
.tbz
to_directory – (
str
) Path to the target directorycreate_dir – (
bool
) Switch to control the creation of a subdirectory withinto_directory
named for the filename of the compressed file. (Default:False
)
- class watchmaker.managers.platform.LinuxPlatformManager(system_params, *args, **kwargs)[source]
Bases:
PlatformManagerBase
Base class for Linux Platforms.
Serves as a foundational class to keep OS consistency.
- class watchmaker.managers.platform.WindowsPlatformManager(system_params, *args, **kwargs)[source]
Bases:
PlatformManagerBase
Base class for Windows Platform.
Serves as a foundational class to keep OS consistency.
watchmaker.managers.worker_manager
Watchmaker workers manager.
- class watchmaker.managers.worker_manager.WorkersManagerBase(system_params, workers, *args, **kwargs)[source]
Bases:
object
Base class for worker managers.
- Parameters:
system_params – (
dict
) Attributes, mostly file-paths, specific to the system-type (Linux or Windows).workers – (
collections.OrderedDict
) Workers to run and associated configuration data.
- class watchmaker.managers.worker_manager.LinuxWorkersManager(system_params, workers, *args, **kwargs)[source]
Bases:
WorkersManagerBase
Manage the worker cadence for Linux systems.
- class watchmaker.managers.worker_manager.WindowsWorkersManager(system_params, workers, *args, **kwargs)[source]
Bases:
WorkersManagerBase
Manage the worker cadence for Windows systems.
watchmaker.workers
Watchmaker workers module.
watchmaker.workers.base
Watchmaker base worker.
watchmaker.workers.salt
Watchmaker salt worker.
- class watchmaker.workers.salt.SaltBase(*args, **kwargs)[source]
Bases:
WorkerBase
,PlatformManagerBase
Cross-platform worker for running salt.
- Parameters:
salt_debug_log – (
list
) Filesystem path to a file where the salt debug output should be saved. When unset, the salt debug log is saved to the Watchmaker log directory. (Default:''
)salt_content – (
str
) URL to a salt content archive (zip file) that will be uncompressed in the watchmaker salt “srv” directory. This typically is used to create a top.sls file and to populate salt’s file_roots. (Default:''
)Linux:
/srv/watchmaker/salt
Windows:
C:\Watchmaker\Salt\srv
salt_content_path – (
str
) Used in conjunction with the “salt_content” arg. Glob pattern for the location of salt content files inside the provided salt_content archive. To be used when salt content files are located within a sub-path of the archive, rather than at its top-level. Multiple paths matching the given pattern will result in error. E.g.salt_content_path='*/'
(Default:''
)salt_states – (
str
) Comma-separated string of salt states to execute. When “highstate” is included with additional states, “highstate” runs first, then the other states. Accepts two special keywords (case-insensitive): (Default:'highstate'
)none
: Do not apply any salt states.highstate
: Apply the salt “highstate”.
exclude_states – (
str
) Comma-separated string of states to exclude from execution. (Default:''
)user_formulas – (
dict
) Map of formula names and URLs to zip archives of salt formulas. These formulas will be downloaded, extracted, and added to the salt file roots. The zip archive must contain a top-level directory that, itself, contains the actual salt formula. To “overwrite” bundled submodule formulas, make sure the formula name matches the submodule name. (Default:{}
)admin_groups – (
str
) Sets a salt grain that specifies the domain groups that should have root privileges on Linux or admin privileges on Windows. Value must be a colon-separated string. E.g."group1:group2"
(Default:''
)admin_users – (
str
) Sets a salt grain that specifies the domain users that should have root privileges on Linux or admin privileges on Windows. Value must be a colon-separated string. E.g."user1:user2"
(Default:''
)environment – (
str
) Sets a salt grain that specifies the environment in which the system is being built. E.g.dev
,test
,prod
, etc. (Default:''
)ou_path – (
str
) Sets a salt grain that specifies the full DN of the OU where the computer account will be created when joining a domain. E.g."OU=SuperCoolApp,DC=example,DC=com"
(Default:''
)pip_install – (
list
) Python packages to be installed prior to applying the high state. (Default:[]
)pip_args – (
list
) Options to pass to pip when installing packages. (Default:[]
)pip_index – (
str
) URL used for an index by pip. (Default:https://pypi.org/simple
)
- process_states(states, exclude)[source]
Apply salt states but exclude certain states.
- Parameters:
states – (
str
) Comma-separated string of salt states to execute. When “highstate” is included with additional states, “highstate” runs first, then the other states. Accepts two special keywords (case-insensitive):none
: Do not apply any salt states.highstate
: Apply the salt “highstate”.
exclude – (
str
) Comma-separated string of states to exclude from execution.
- class watchmaker.workers.salt.SaltLinux(*args, **kwargs)[source]
Bases:
SaltBase
,LinuxPlatformManager
Run salt on Linux.
- Parameters:
install_method – (
str
) Required. Method to use to install salt. (Default:yum
)yum
: Install salt from an RPM using yum.git
: Install salt from source, using the salt bootstrap.
bootstrap_source – (
str
) URL to the salt bootstrap script. Required ifinstall_method
isgit
. (Default:''
)git_repo – (
str
) URL to the salt git repo. Required ifinstall_method
isgit
. (Default:''
)salt_version – (
str
) A git reference present ingit_repo
, such as a commit or a tag. If not specified, the HEAD of the default branch is used. (Default:''
)
- class watchmaker.workers.salt.SaltWindows(*args, **kwargs)[source]
Bases:
SaltBase
,WindowsPlatformManager
Run salt on Windows.
- Parameters:
watchmaker.workers.yum
Watchmaker yum worker.
- class watchmaker.workers.yum.Yum(*args, **kwargs)[source]
Bases:
WorkerBase
,LinuxPlatformManager
Install yum repos.
- Parameters:
repo_map – (
list
) List of dictionaries containing a map of yum repo files to systems. (Default:[]
)